일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 파이썬
- medium
- SQL
- 구글퀵랩
- dfs
- 니트코드
- 릿코드
- 자연어처리
- heap
- 코드업
- 생성형AI
- Python
- 파이썬기초100제
- GenAI
- two-pointer
- gcp
- 슬라이딩윈도우
- GenerativeAI
- LeetCode
- 리트코드
- Python3
- slidingwindow
- nlp
- Microsoft
- 알고리즘
- 파이썬알고리즘
- codeup
- 투포인터
- stratascratch
- sql코테
Archives
- Today
- Total
Tech for good
[NeetCode 150/String Manipulation,TwoPointer] Valid Palindrome 본문
IT/Computer Science
[NeetCode 150/String Manipulation,TwoPointer] Valid Palindrome
Diana Kang 2025. 2. 21. 12:16https://neetcode.io/problems/is-palindrome
NeetCode
neetcode.io
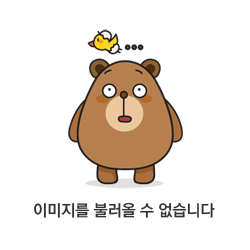
첫번째 방법 - String Manipulation
class Solution:
def isPalindrome(self, s: str) -> bool:
# 1. 알파벳과 숫자만 남기고 소문자로 변환
filtered_s = ''.join(c.lower() for c in s if c.isalnum())
# 2. 문자열을 뒤집어서 원래 문자열과 비교
return filtered_s == filtered_s[::-1]
- isalnum() -> 문자열이 문자 혹은 숫자로 되어있으면 참 리턴, 아니면 거짓 리턴
- 시간 복잡도
- 문자열 필터링: O(n)
- 문자열 비교: O(n)
- 총 시간복잡도: O(n) (입력 문자열 길이 에 대해 선형 시간)
두번째 방법 - Two Pointers
투 포인터 (Two Pointers) 방식의 핵심 개념
- 왼쪽 포인터 (left): 문자열의 시작에서 오른쪽으로 이동
- 오른쪽 포인터 (right): 문자열의 끝에서 왼쪽으로 이동
- 목표: 두 포인터가 서로 만날 때까지 진행하면서 알파벳과 숫자만 비교
- 필요한 작업: 공백, 특수문자 등 비알파벳/비숫자 문자는 건너뛴다
class Solution:
def isPalindrome(self, s: str) -> bool:
left, right = 0, len(s) - 1
while left < right: # left 포인터와 right 포인터가 서로 교차하지 않을 때까지 반복
# 왼쪽 포인터 이동 (알파벳/숫자가 아닐 경우)
while left < right and not s[left].isalnum():
left += 1 # left를 오른쪽으로 이동시킴 -> 즉, 공백, 특수문자, 마침표 등을 건너뜀
# 오른쪽 포인터 이동 (알파벳/숫자가 아닐 경우)
while left < right and not s[right].isalnum():
right -= 1 # right를 왼쪽으로 이동시킴 -> 즉, 공백, 특수문자, 마침표 등을 건너뜀
# 소문자로 변환 후 비교
if s[left].lower() != s[right].lower():
return False
left += 1
right -= 1
return True
- 시간/공간 복잡도
- 공간복잡도: O(1) → 추가적인 문자열을 생성하지 않으므로 메모리 절약
- 시간복잡도: O(n) → 문자열을 한 번만 순회
'IT > Computer Science' 카테고리의 다른 글
[Leetcode/TwoPointer] 2825. Make String a Subsequence Using Cyclic Increments (0) | 2025.02.22 |
---|---|
[Leetcode/TwoPointer] 345. Reverse Vowels of a String (0) | 2025.02.21 |
[Leetcode/TwoPointer+Queue (Greedy)] 2149. Rearrange Array Elements by Sign (0) | 2025.02.21 |
[Leetcode/TwoPointer] 443. String Compression (0) | 2025.02.21 |
[Leetcode/TwoPointer] 977. Squares of a Sorted Array (0) | 2025.02.21 |