일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 릿코드
- heap
- Python
- 알고리즘
- codeup
- 파이썬기초100제
- Python3
- medium
- 리트코드
- GenerativeAI
- dfs
- GenAI
- 슬라이딩윈도우
- 생성형AI
- gcp
- 니트코드
- stratascratch
- slidingwindow
- 파이썬알고리즘
- 코드업
- two-pointer
- 투포인터
- 자연어처리
- nlp
- 파이썬
- Microsoft
- 구글퀵랩
- sql코테
- LeetCode
- SQL
Archives
- Today
- Total
Tech for good
[Leetcode/Heap] 2558. Take Gifts From the Richest Pile 본문
IT/Computer Science
[Leetcode/Heap] 2558. Take Gifts From the Richest Pile
Diana Kang 2025. 4. 4. 22:57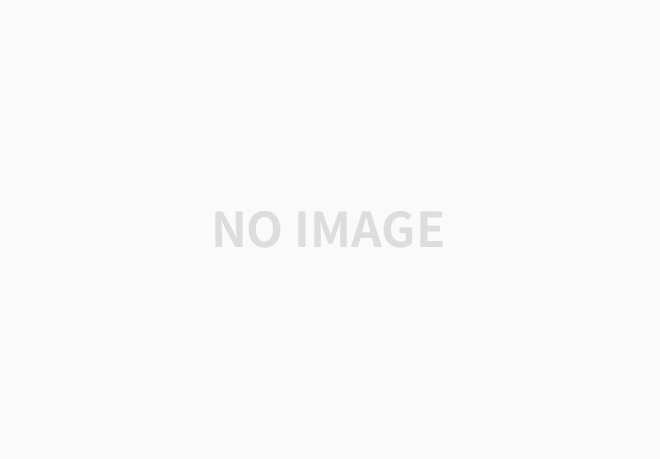
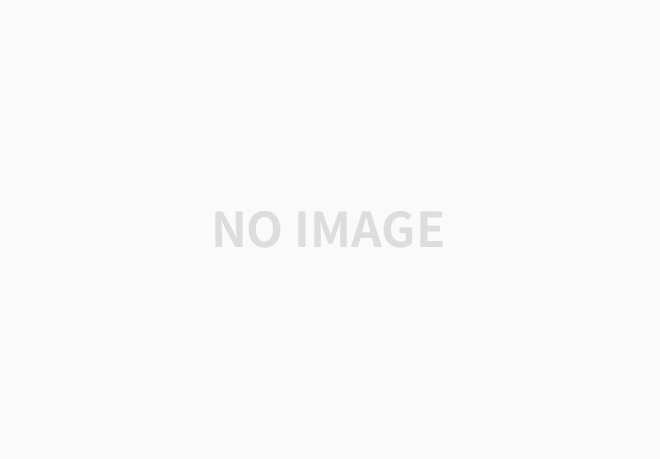
Example 1:
gifts = [25, 64, 9, 4, 100], k = 4
[25, 64, 9, 4, 100]
-> (1회) [25, 64, 9, 4, 10]
-> (2회) [25, 8, 9, 4, 10]
-> (3회) [5, 8, 9, 4, 10]
-> (4회) [5, 8, 9, 4, 3] (floor or the square root - floor: 내림)
5 + 8 + 9 + 4 + 3 = 29
Follow these steps:
- Pick the largest pile of gifts.
- Replace it with the floor of its square root.
- Repeat this for k seconds.
🔧 Best Tool for This?
We need to efficiently get the largest number repeatedly — that's a classic use case for a max heap.
BUT: Python’s heapq module only supports min-heaps, so we simulate a max-heap by inserting negative numbers.
class Solution:
def pickGifts(self, gifts: List[int], k: int) -> int:
# Step 1: Convert to max-heap by inserting negative values
max_heap = [-g for g in gifts]
heapq.heapify(max_heap)
# Step 2: Perform the operation k times
for _ in range(k):
# Pop the largest (most negative = smallest negative)
largest = -heapq.heappop(max_heap)
reduced = math.isqrt(largest) # floor(sqrt(x))
heapq.heappush(max_heap, -reduced)
# Step 3: Sum up the remaining gifts (convert back to positive)
return -sum(max_heap)
💡 Notes:
- math.isqrt(x) is a fast and accurate way to do floor(sqrt(x)) for integers.
- Using a heap makes this efficient even when the list is long or k is big.
📚 math.isqrt(x) explained:
math.isqrt(x) is a function in Python that returns the integer (floor) square root of x.
It gives you the largest integer whose square is less than or equal to x.
import math
print(math.isqrt(10)) # 3, because 3^2 = 9 (and 4^2 = 16 > 10)
print(math.isqrt(25)) # 5, because 5^2 = 25
print(math.isqrt(100)) # 10
print(math.isqrt(1)) # 1
'IT > Computer Science' 카테고리의 다른 글
[Leetcode/Heap] 3264. Final Array State After K Multiplication Operations I (0) | 2025.04.07 |
---|---|
[Leetcode/Sort, Heap] 2974. Minimum Number Game (0) | 2025.04.06 |
[Leetcode/Heap] 2099. Find Subsequence of Length K With the Largest Sum (0) | 2025.04.04 |
[Leetcode/Heap] 1464. Maximum Product of Two Elements in an Array (0) | 2025.03.31 |
[Neetcode/Heap] K Closest Points to Origin (0) | 2025.03.31 |